How to Debug C Code in Xcode
Feb. 9, 2017 | smlee36 | #seneca, #c, #beginner, #xcode, #debug, #Raphael
This is a ultra simple tutorial for debugging c program in Xcode.
TOC
Case Study
Sample Program
Run Xcode and Open your progect. If you don't know how to make a project,
read this post first.
A program instruction is here.
- input number between 1 ~ 10
- If input number is invalid (out of range), print a error message and input again until the number is valid.
- this is a sample code for the instruction.
// An ultra simple debugging example
#include <stdio.h>
int main() {
int num;
printf("Enter your number (1-10): ");
scanf("%d", &num);
while (num < 1 || 10 < num) {
printf("Input number should be 1 - 10.");
printf("Enter your number again (1-10): ");
}
printf("Your number is %d.\n", num);
return 0;
}
- Now run 'Product > Run' (⌘+R), the program. The 'Enter your number (1-10): ' message is displayed on the center-bottom pane. Enter '5' . Then the program will be end normally.
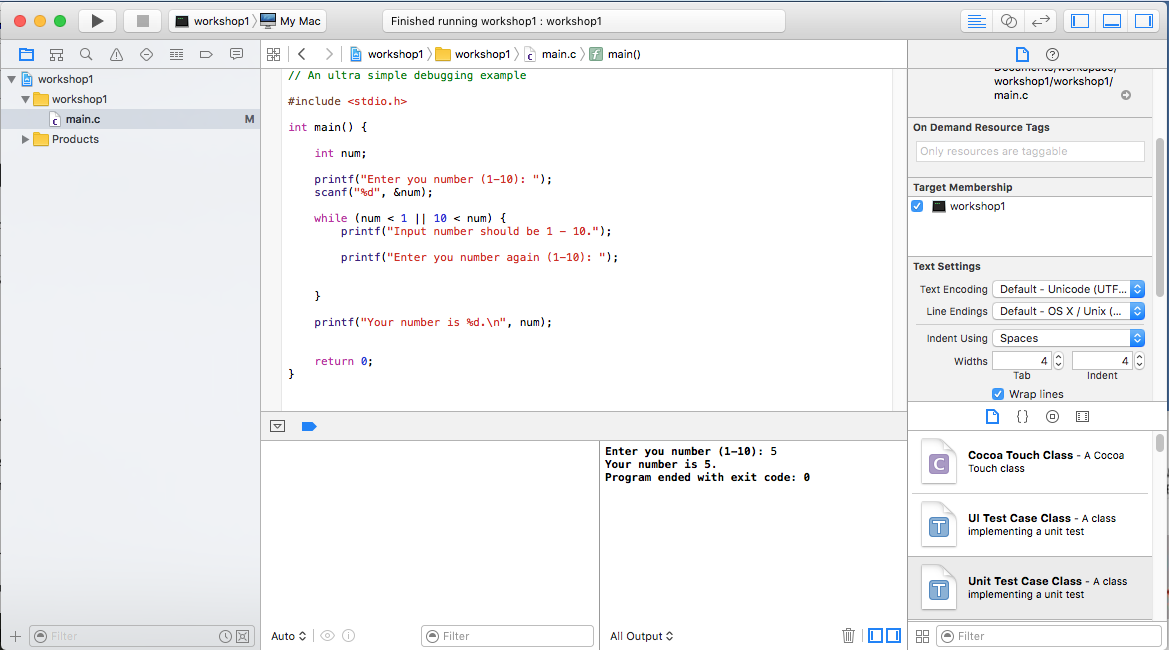
- Now run the program again. Enter '20' . The program shows an error message continuously. Stop the program using 'Product > Stop' (⌘+.)
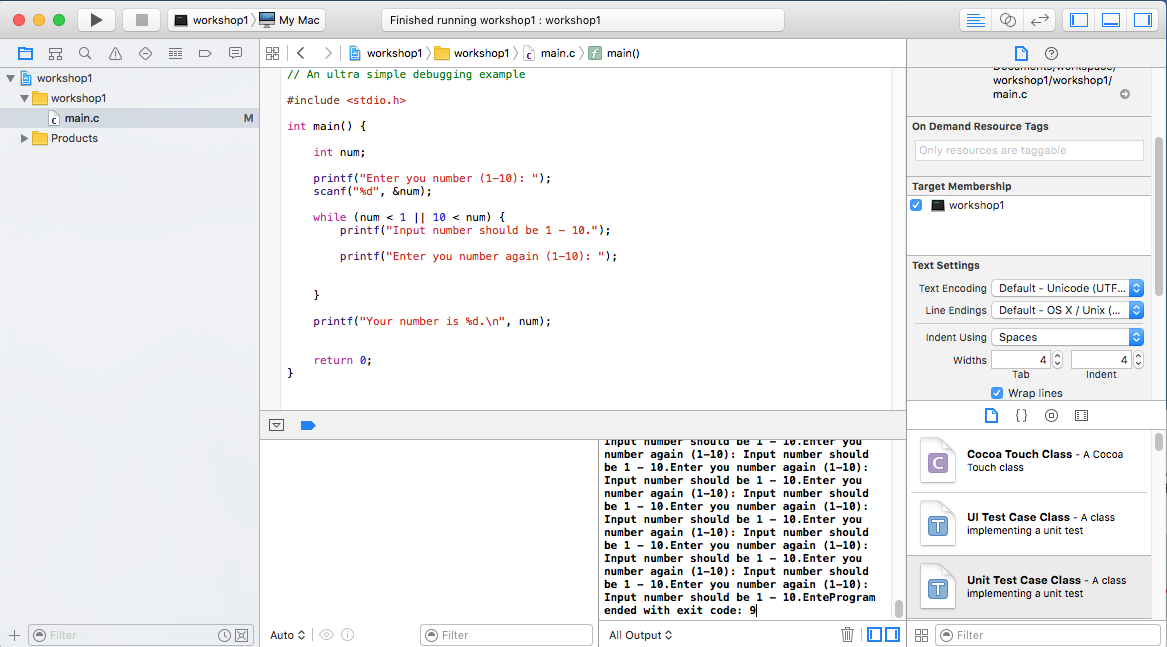
Debug the Program
Marking break points
- First of all, select some position. If you don't know where you should choose, select the first line. Press left mouse button at the left-side empty area of center window. You can see a blue break point label.
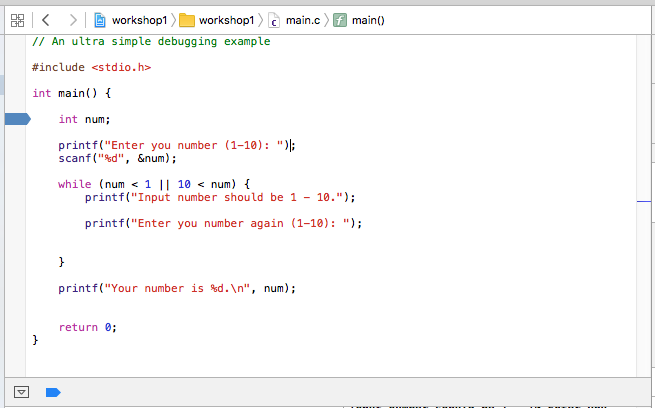
- Now run 'Product > Run' (⌘+R), the program. Enter an invalid number '11' . Then a green current position marker will be displayed. It is a marker to indicate in where C compiler is doing it's job now.
- Press 'F6' then the process marker will proceed line by line.
- When the process marker locate at 'Enter your number again' message, the marker will go back to the beginning of while loop instead of going 'Your number....' line.
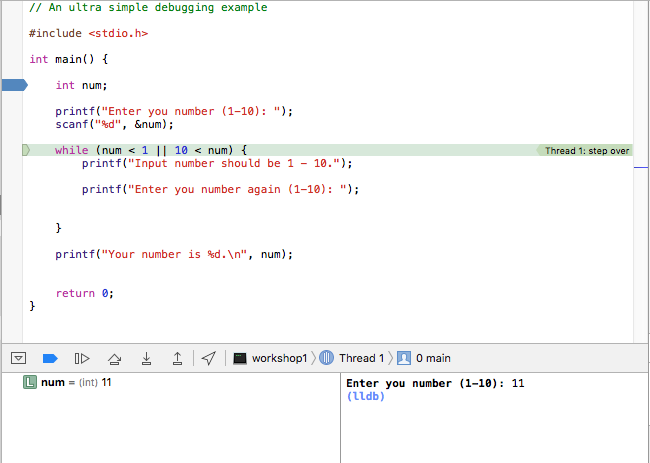
So, we can realize something is needed after 'Enter your number again..' to stop infinite loop.
Insert this statement into after 'Enter your number again' line.
scanf("%d", &num);
- Now the program works well as we expected.
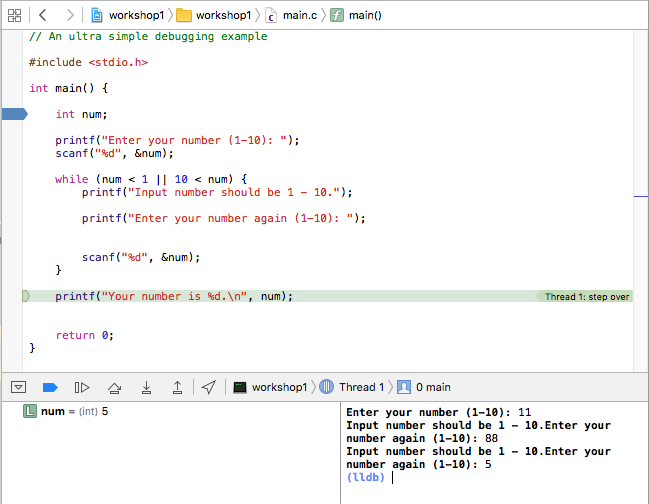