How to Debug C Code in a Visual Studio
Feb. 9, 2017 | smlee36 | #seneca, #c, #beginner, #visualstudio, #debug, #Abdi
This is an ultra simple tutorial for debugging c program in a Visual Studio.
TOC
Case Study
Sample Program
Run Visual Studio and Open your progect. If you don't know how to make a project,
read this post first.
A program instruction is here.
- input number between 1 ~ 10
- If input number is invalid (out of range), print a error message and input again until the number is valid.
- this is a sample code for the instruction.
// An ultra simple debugging example
#include <stdio.h>
int main() {
int num;
printf("Enter your number (1-10): ");
scanf("%d", &num);
while (num < 1 || 10 < num) {
printf("Input number should be 1 - 10.");
printf("Enter your number again (1-10): ");
}
printf("Your number is %d.\n", num);
return 0;
}
- Now run 'DEBUG > Start without debuging' (Ctrl + F5), the program. The 'Enter your number (1-10): ' message is displayed on the center-bottom pane. Enter '5' . Then the program will be end normally.
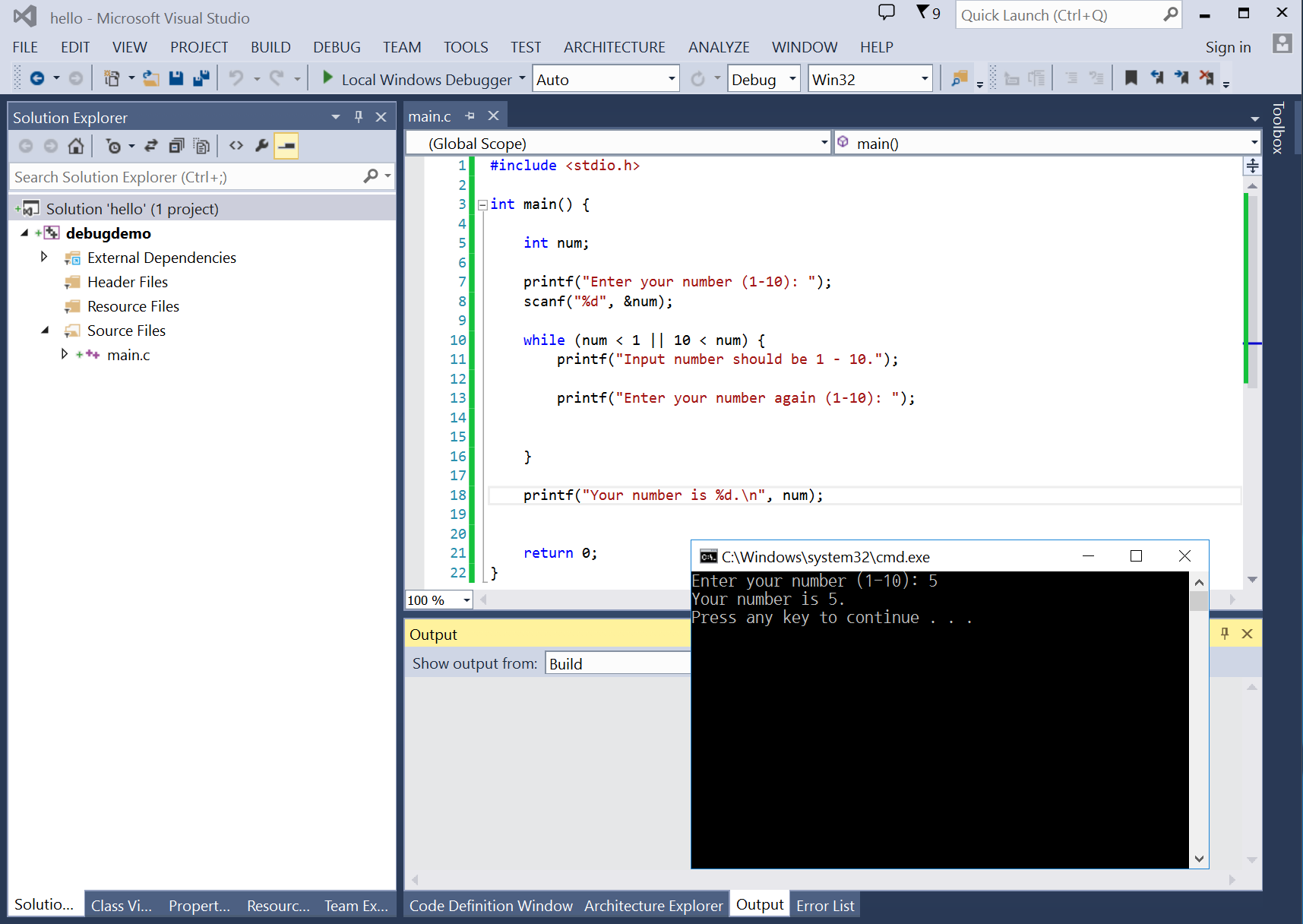
- Now run the program again. Enter '11' . The program shows an error message continuously.
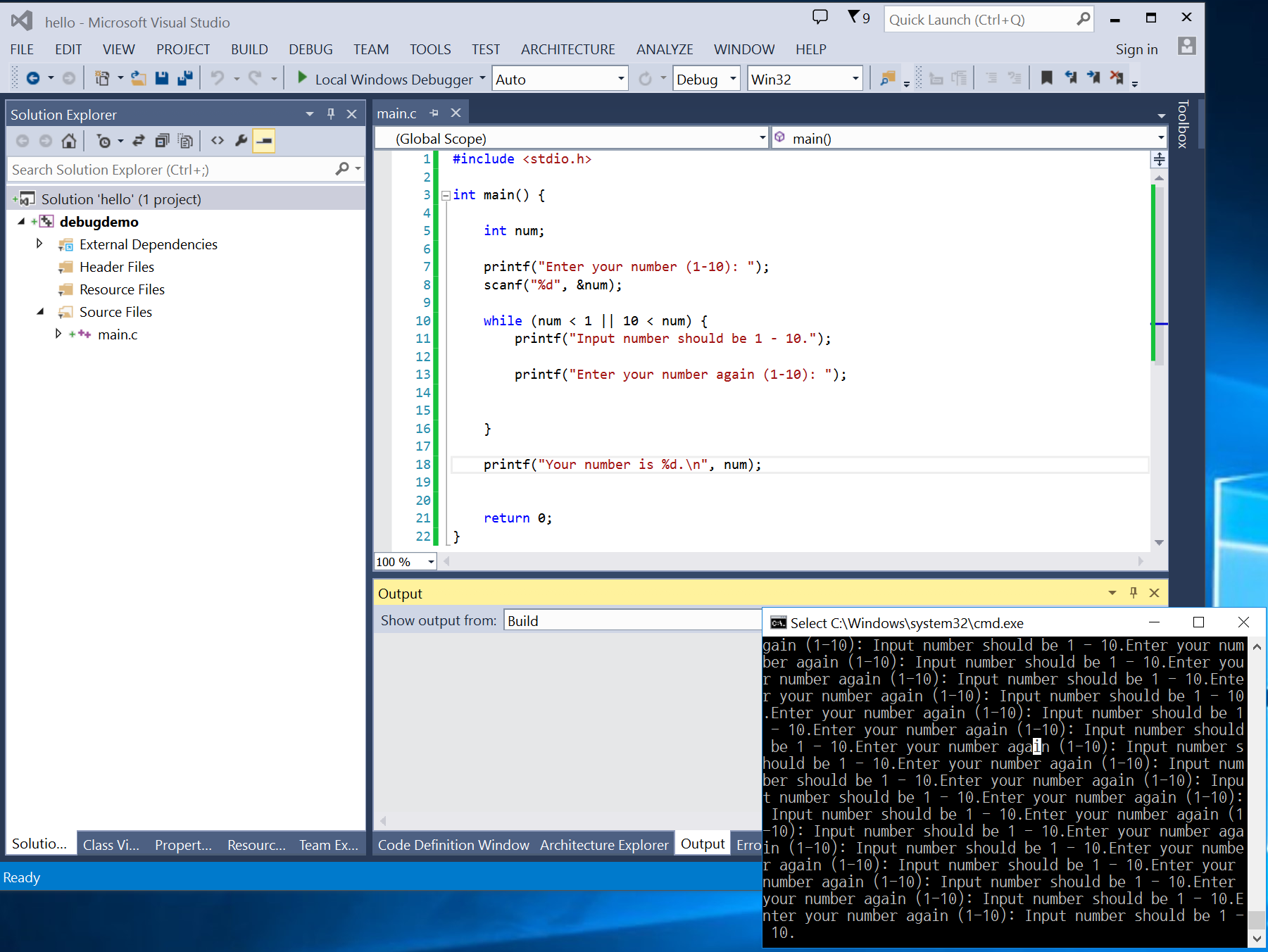
Debug the Program
Marking break points
- First of all, select some position. If you don't know where you should choose, select the first line (line 7). Press a RIGHT MOUSE BUTTON at the variable on line 7. Choose 'Insert Breakpoint', then a red break point mark will be shown.
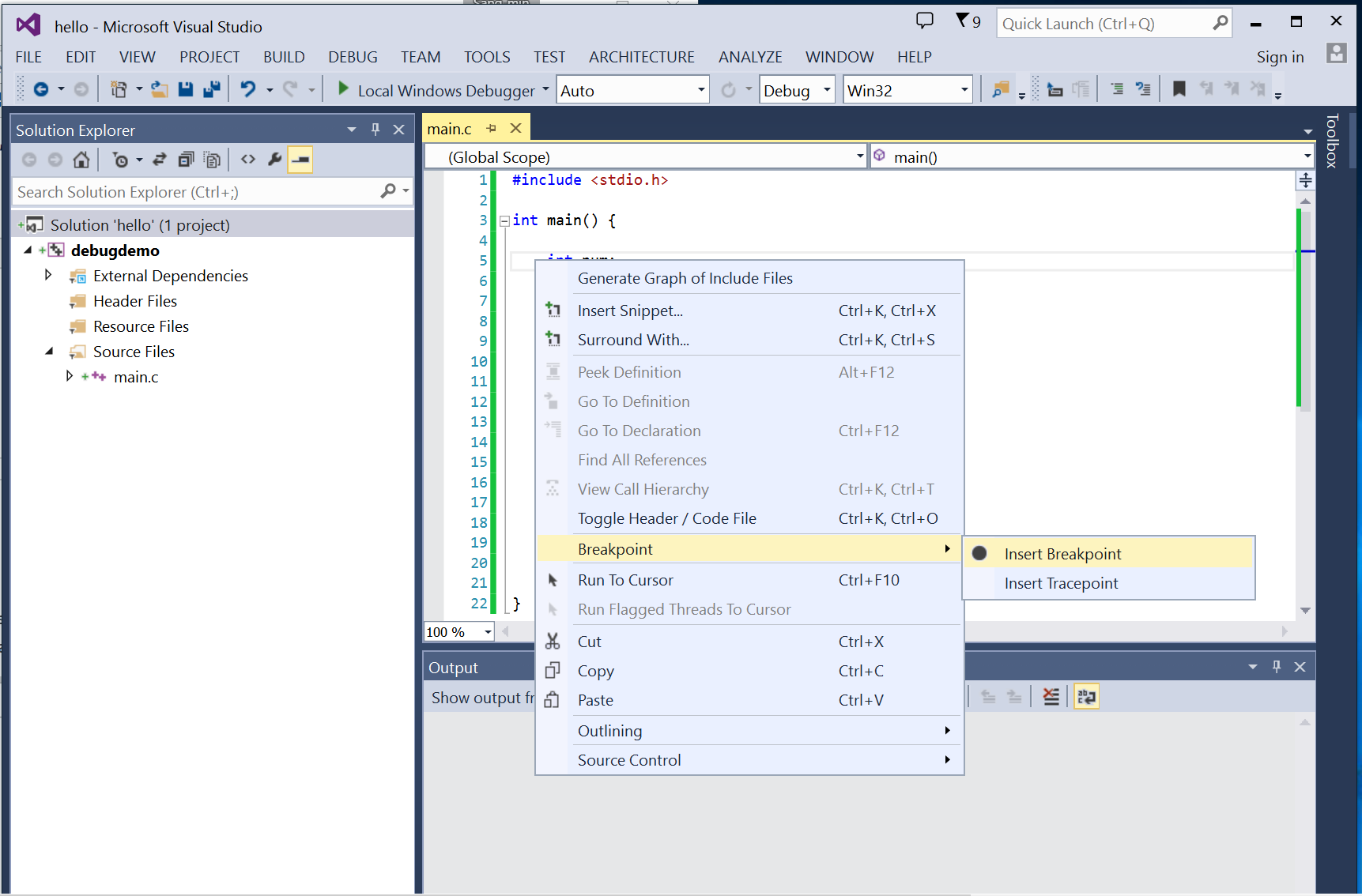
- Now run 'DEBUG > Start with debuging' (Ctrl + F5) the program. Enter an invalid number '11' . Then a yellow current position arrow will be displayed at line 7. It is a marker to indicate in where C compiler is doing it's job now.
- Press 'F11'(Step into) then the process marker will proceed line by line.
- When the process marker locate at 'Enter your number again' message, the marker will go back to the beginning of while loop instead of going line 18.
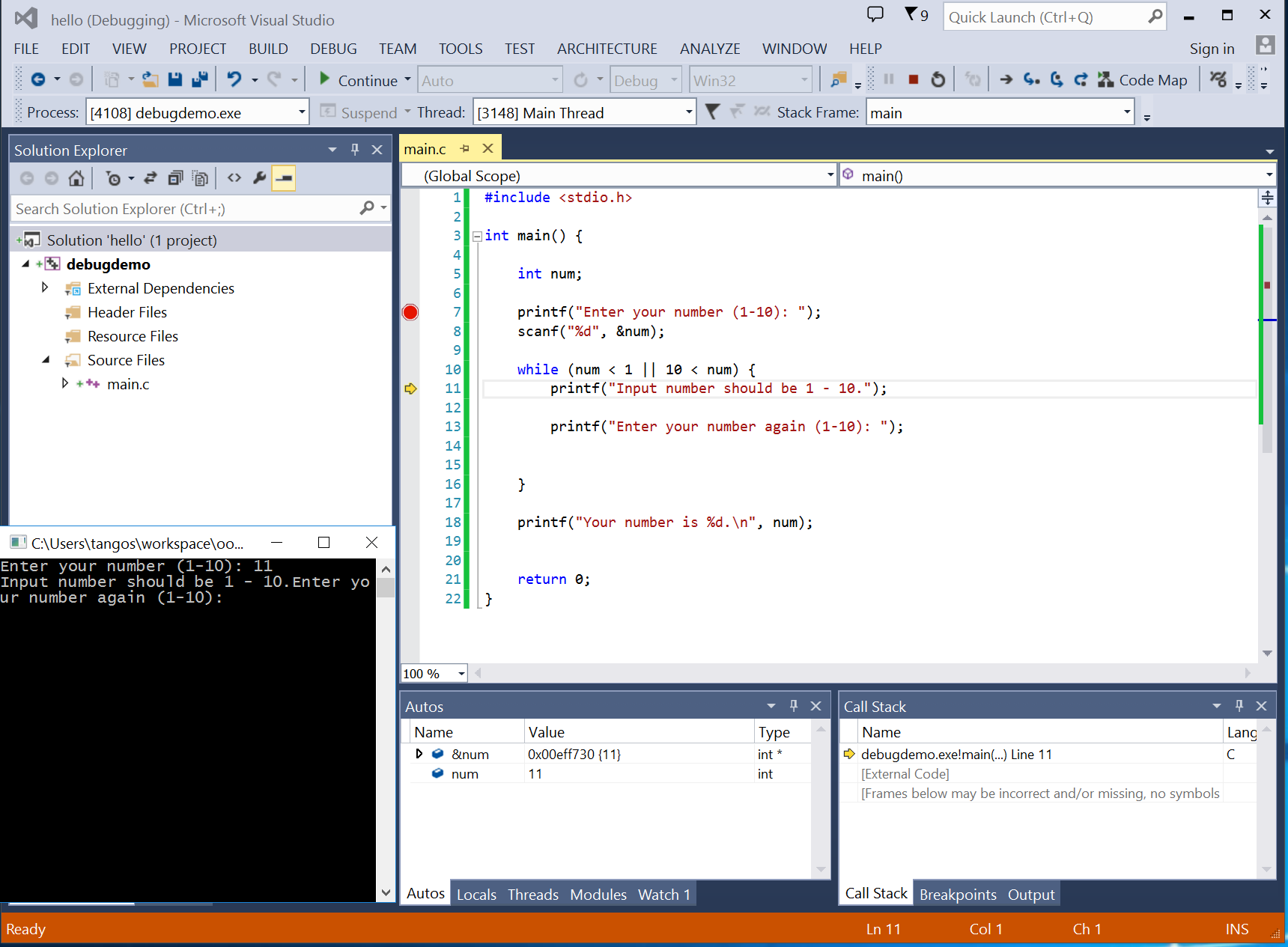
So, we can realize something is needed after line 13 to stop infinite loop.
Insert this statement into after line 14.
scanf("%d", &num);
As described above, developer can see the exact position of compiler in progress.